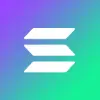
Token Transfer with Anchor
Imports and Attributes: Imports from Anchor prelude. #[program] marks the start of the Solana program. Module definition for token transfer. State Struct: #[state] for the program's state. Struct declaration for MyProgram. Implementation Block: Methods associated with MyProgram. Token Transfer Method: transfer_tokens function for token transfers. Takes transaction context and token amount. CPI Accounts Handling: Creates Transfer struct with account references. Cloning CPI Program: Clones the token program account. Creating CPI Context: Combines token program and CPI accounts. Token Transfer Invocation: Invokes token transfer function with CPI context. Return: Returns Ok(()) for successful transfer.

CAVYAR AI
CAVYAR AI is in its earliest stages of development and the quality of generated descriptions will improve massively over time. At this stage, there is a risk of false information being generated.
The provided code snippet is a Solana smart contract written using the Anchor framework, specifically designed to transfer tokens between accounts. The main functionality is encapsulated in the transfer_tokens
function.
Key Components Explained:
-
Imports and Anchor Prelude: The
use anchor_lang::prelude::*;
import brings in necessary components from the Anchor framework, which simplifies Solana smart contract development. -
Program Declaration: The
#[program]
attribute andmod token_transfer
define the smart contract module. This is where the logic of the contract is encapsulated. -
State Struct: The
#[state]
attribute andpub struct MyProgram {}
define the state of the smart contract. In this case, the state is empty, indicating that no persistent state is stored in this contract. -
Token Transfer Method: The
transfer_tokens
function is the core of this smart contract. It takes a context (ctx
) and an amount of lamports (tokens) to transfer. The function performs a Cross-Program Invocation (CPI) to the Solana Token Program to transfer tokens from one account to another.-
Context and Accounts: The
Context<'_, '_, '_, 'info, TransferTokens>
parameter is a generic type that holds references to the accounts needed for the token transfer. These accounts include the sender, receiver, and authority accounts, among others necessary for the operation. -
CPI Accounts Handling: The
Transfer
struct is created with account references from the context. This struct represents the accounts involved in the token transfer operation. -
Cloning CPI Program: The token program account is cloned from the context. This account represents the Solana Token Program, which is called to perform the actual token transfer.
-
Creating CPI Context: A
CpiContext
is created with the token program and theTransfer
accounts. This context is used to invoke the token transfer operation. -
Token Transfer Invocation: The
token::transfer(cpi_ctx, lamports)?;
line invokes the token transfer operation of the Solana Token Program. The?
operator is used for error handling, propagating any errors encountered during the transfer.
-
-
Return: The function returns
Ok(())
upon successful completion of the token transfer, indicating that the operation was successful without any errors.
Conclusion: This smart contract function demonstrates how to perform a token transfer on the Solana blockchain using the Anchor framework. It showcases the use of CPI (Cross-Program Invocation) to interact with the Solana Token Program, a common pattern for Solana smart contracts that need to perform operations involving SPL tokens.
Ask

Soon™