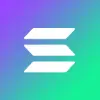
Retrieve Solana Token Balance with JavaScript
Fetch Solana token balance using JavaScript with '@solana/web3.js', providing connection details, public key, and token mint address.

CAVYAR AI
CAVYAR AI is in its earliest stages of development and the quality of generated descriptions will improve massively over time. At this stage, there is a risk of false information being generated.
This code snippet is designed to retrieve the balance of a specific token for a given public key on the Solana blockchain using JavaScript and the @solana/web3.js
library. It defines an asynchronous function getTokenBalance
that takes a Connection
object, a PublicKey
for the wallet of interest, and a PublicKey
for the token mint address. It uses these to create a Token
object and calls its getBalance
method to fetch the token balance. The balance is then logged to the console.
Detailed Explanation:
-
Importing Necessary Classes: The snippet starts by importing
Connection
,PublicKey
, andToken
classes from@solana/web3.js
. These are essential for interacting with the Solana blockchain.Connection
: Used to establish a connection with the Solana blockchain.PublicKey
: Represents public keys on the Solana blockchain.Token
: A class representing a token on the Solana blockchain, allowing for operations such as fetching balances.
-
The
getTokenBalance
Function:- It is an asynchronous function, indicating operations within it may not complete instantly.
- Takes three parameters:
connection
(an instance ofConnection
),publicKey
(instance ofPublicKey
for the wallet), andtokenMintAddress
(instance ofPublicKey
for the token's mint address). - Inside, it creates a new
Token
instance using the providedconnection
,tokenMintAddress
, andnull
for the programId (assuming the default token program). - It then awaits the result of the
getBalance
method called on theToken
instance, passing thepublicKey
. - Finally, it returns the balance.
-
Establishing a Connection:
- A new
Connection
is created with the URL of the Solana mainnet beta cluster and a commitment level of "processed". This sets the level of confirmation the node should consider a transaction to be finalized.
- A new
-
Defining Public Keys:
- Two
PublicKey
instances are created, one for the user's public key and another for the token mint address. These are placeholders and should be replaced with actual public key strings.
- Two
-
Executing the Function:
- The
getTokenBalance
function is called with theconnection
,publicKey
, andtokenMintAddress
. It returns a promise that resolves with the balance. .then()
is used to handle the resolved promise, logging the balance to the console..catch()
is used to catch and log any errors that occur during the execution.
- The
Conclusion: This code is a straightforward example of how to fetch a token's balance for a specific wallet on the Solana blockchain using JavaScript. It leverages the @solana/web3.js
library to interact with the blockchain, making it a useful snippet for developers working on Solana-based applications requiring balance checks.
Ask

Soon™